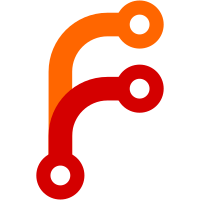
This is the beta version of the Morse Converter for the web. It is a complete rewrite and now a full webapp using Polymer. It supports all the features of the desktop and the android version.
522 lines
12 KiB
CoffeeScript
522 lines
12 KiB
CoffeeScript
getNormalMorseDecoded = (input) ->
|
|
# Well, this shouldn't happen...
|
|
if !(input)
|
|
return null
|
|
|
|
# remove trailing whitspaces
|
|
if input.charAt input.length is " "
|
|
input.substring 0, input.length - 1
|
|
|
|
input = input.toUpperCase()
|
|
output = ""
|
|
|
|
# Return special signs
|
|
if input is "LETTERSPACE"
|
|
return " "
|
|
else if input is "END OF WORK"
|
|
return "...-.-"
|
|
else if input is "ERROR"
|
|
return "........"
|
|
else if input is "STARTING SIGNAL"
|
|
return "-.-.-"
|
|
else if input is "ENDING SIGNAL"
|
|
return ".-.-."
|
|
else if input is "UNDERSTOOD"
|
|
return "...-."
|
|
else if input is "WAIT"
|
|
return ".-..."
|
|
else if input is "SOS"
|
|
return "...---..."
|
|
else if input is "LETTER SPACE"
|
|
return " "
|
|
else if input is "WORD SPACE"
|
|
return " "
|
|
else # the converting part
|
|
|
|
while input.length > 0
|
|
|
|
if input.charAt(0) is " "
|
|
if output.charAt(output.length) is " "
|
|
output = output.substring 0, output.length - 1
|
|
output += " "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "\n"
|
|
output += "<br>"
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "A"
|
|
output += ".- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "B"
|
|
output += "-... "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "C"
|
|
output += "-.-. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "D"
|
|
output += "-.. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "E"
|
|
output += ". "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "F"
|
|
output += "..-. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "G"
|
|
output += "--. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "H"
|
|
output += ".... "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "I"
|
|
output += ".. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "J"
|
|
output += ".--- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "K"
|
|
output += "-.- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "L"
|
|
output += ".-.. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "M"
|
|
output += "-- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "N"
|
|
output += "-. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "O"
|
|
output += "--- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "P"
|
|
output += ".--. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "Q"
|
|
output += "--.- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "R"
|
|
output += ".-. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "S"
|
|
output += "... "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "T"
|
|
output += "- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "U"
|
|
output += "..- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "V"
|
|
output += "...- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "W"
|
|
output += ".-- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "X"
|
|
output += "-..- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "Y"
|
|
output += "-.-- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "Z"
|
|
output += "--.. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "0"
|
|
output += "----- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "1"
|
|
output += ".---- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "2"
|
|
output += "..--- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "3"
|
|
output += "...-- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "4"
|
|
output += "....- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "5"
|
|
output += "..... "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "6"
|
|
output += "-.... "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "7"
|
|
output += "--... "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "8"
|
|
output += "---.. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "9"
|
|
output += "----. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "Ä"
|
|
output += ".-.- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "Ö"
|
|
output += "---. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "Ü"
|
|
output += "..-- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "ß"
|
|
output += "...--... "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "."
|
|
output += ".-.-.- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is ","
|
|
output += "--..-- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is ":"
|
|
output += "---... "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is ""
|
|
output += "-.-.-. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "?"
|
|
output += "..--.. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "!"
|
|
output += "-.-.-- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "-"
|
|
output += "-....- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "_"
|
|
output += "..--.- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "("
|
|
output += "-.--. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is ")"
|
|
output += "-.--.- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "="
|
|
output += "-...- "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "+"
|
|
output += ".-.-. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "/"
|
|
output += "-..-. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "@"
|
|
output += ".--.-. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "'"
|
|
output += ".----. "
|
|
input = input.substring 1
|
|
|
|
else if input.charAt(0) is "$"
|
|
output += "...-..- "
|
|
input = input.substring 1
|
|
|
|
else
|
|
return "Code not listed or wrong."
|
|
|
|
if output.charAt(output.length - 1) is " "
|
|
output = output.substring 0, output.length - 1
|
|
|
|
return output
|
|
|
|
getNormalMorseEncoded = (input) ->
|
|
# Well, this shouldn't happen...
|
|
if !(input) then return null
|
|
|
|
# remove trailing whitspaces
|
|
while input.charAt(input.length - 1) is " "
|
|
input = input.substring 0, input.length - 1
|
|
|
|
input = input.toUpperCase()
|
|
|
|
# remove all trailing new lines
|
|
while input.charAt(input.length - 1) is "\n"
|
|
input = input.substring 0, input.length - 1
|
|
|
|
# add one letterspace to the input
|
|
input += " "
|
|
|
|
inputToSign = input
|
|
output = ""
|
|
|
|
while inputToSign isnt " "
|
|
|
|
d = 0
|
|
signFull = true
|
|
sign = ""
|
|
|
|
while signFull
|
|
|
|
if inputToSign.substring(d, d + 7) is " " or
|
|
inputToSign.substring(d, d + 3) is " " or
|
|
inputToSign.charAt(0) is "\n"
|
|
|
|
if d is 0
|
|
|
|
if inputToSign.substring(0, 7) is " "
|
|
output += " "
|
|
inputToSign = inputToSign.substring 7, inputToSign.length
|
|
|
|
if inputToSign.substring(0, 3) is " "
|
|
inputToSign = inputToSign.substring 3, inputToSign.length
|
|
|
|
if inputToSign.charAt(0) is "\n"
|
|
output += "<br>"
|
|
inputToSign = inputToSign.substring 1, inputToSign.length
|
|
|
|
else
|
|
sign = inputToSign.substring 0, d
|
|
inputToSign = inputToSign.substring d, inputToSign.length
|
|
signFull = false
|
|
|
|
else
|
|
d++
|
|
|
|
if sign is ".-"
|
|
output += "A"
|
|
|
|
else if sign is "-..."
|
|
output += "B"
|
|
|
|
else if sign is "-.-."
|
|
output += "C"
|
|
|
|
else if sign is "-.."
|
|
output += "D"
|
|
|
|
else if sign is "."
|
|
output += "E"
|
|
|
|
else if sign is "..-."
|
|
output += "F"
|
|
|
|
else if sign is "--."
|
|
output += "G"
|
|
|
|
else if sign is "...."
|
|
output += "H"
|
|
|
|
else if sign is ".."
|
|
output += "I"
|
|
|
|
else if sign is ".---"
|
|
output += "J"
|
|
|
|
else if sign is "-.-"
|
|
output += "K"
|
|
|
|
else if sign is ".-.."
|
|
output += "L"
|
|
|
|
else if sign is "--"
|
|
output += "M"
|
|
|
|
else if sign is "-."
|
|
output += "N"
|
|
|
|
else if sign is "---"
|
|
output += "O"
|
|
|
|
else if sign is ".--."
|
|
output += "P"
|
|
|
|
else if sign is "--.-"
|
|
output += "Q"
|
|
|
|
else if sign is ".-."
|
|
output += "R"
|
|
|
|
else if sign is "..."
|
|
output += "S"
|
|
|
|
else if sign is "-"
|
|
output += "T"
|
|
|
|
else if sign is "..-"
|
|
output += "U"
|
|
|
|
else if sign is "...-"
|
|
output += "V"
|
|
|
|
else if sign is ".--"
|
|
output += "W"
|
|
|
|
else if sign is "-..-"
|
|
output += "X"
|
|
|
|
else if sign is "-.--"
|
|
output += "Y"
|
|
|
|
else if sign is "--.."
|
|
output += "Z"
|
|
|
|
else if sign is "-----"
|
|
output += "0"
|
|
|
|
else if sign is ".----"
|
|
output += "1"
|
|
|
|
else if sign is "..---"
|
|
output += "2"
|
|
|
|
else if sign is "...--"
|
|
output += "3"
|
|
|
|
else if sign is "....-"
|
|
output += "4"
|
|
|
|
else if sign is "....."
|
|
output += "5"
|
|
|
|
else if sign is "-...."
|
|
output += "6"
|
|
|
|
else if sign is "--..."
|
|
output += "7"
|
|
|
|
else if sign is "---.."
|
|
output += "8"
|
|
|
|
else if sign is "----."
|
|
output += "9"
|
|
|
|
else if sign is ".-.-"
|
|
output += "Ä"
|
|
|
|
else if sign is "---."
|
|
output += "Ö"
|
|
|
|
else if sign is "..--"
|
|
output += "Ü"
|
|
|
|
else if sign is "...--..."
|
|
output += "ß"
|
|
|
|
else if sign is "----"
|
|
output += "CH"
|
|
|
|
else if sign is ".-.-.-"
|
|
output += "."
|
|
|
|
else if sign is "--..--"
|
|
output += ","
|
|
|
|
else if sign is "---..."
|
|
output += ":"
|
|
|
|
else if sign is "-.-.-."
|
|
output += ";"
|
|
|
|
else if sign is "..--.."
|
|
output += "?"
|
|
|
|
else if sign is "-.-.--"
|
|
output += "!"
|
|
|
|
else if sign is "-....-"
|
|
output += "-"
|
|
|
|
else if sign is "..--.-"
|
|
output += "_"
|
|
|
|
else if sign is "-.--."
|
|
output += "("
|
|
|
|
else if sign is "-.--.-"
|
|
output += ")"
|
|
|
|
else if sign is ".----."
|
|
output += "'"
|
|
|
|
else if sign is "-...-"
|
|
output += "="
|
|
|
|
else if sign is ".-.-."
|
|
output += "+"
|
|
|
|
else if sign is "-..-."
|
|
output += "/"
|
|
|
|
else if sign is ".--.-."
|
|
output += "@"
|
|
|
|
else if sign is "-.-.-"
|
|
output += "Begin of the signal"
|
|
|
|
else if sign is "-...-"
|
|
output += "Wait"
|
|
|
|
else if sign is "...-."
|
|
output += "Understood"
|
|
|
|
else if sign is "...-.-"
|
|
output += "End of work"
|
|
|
|
else if sign is "...---..."
|
|
output += "SOS"
|
|
|
|
else if sign is "........"
|
|
output += "Error"
|
|
|
|
else
|
|
return "Code not listed or wrong."
|
|
|
|
return output
|